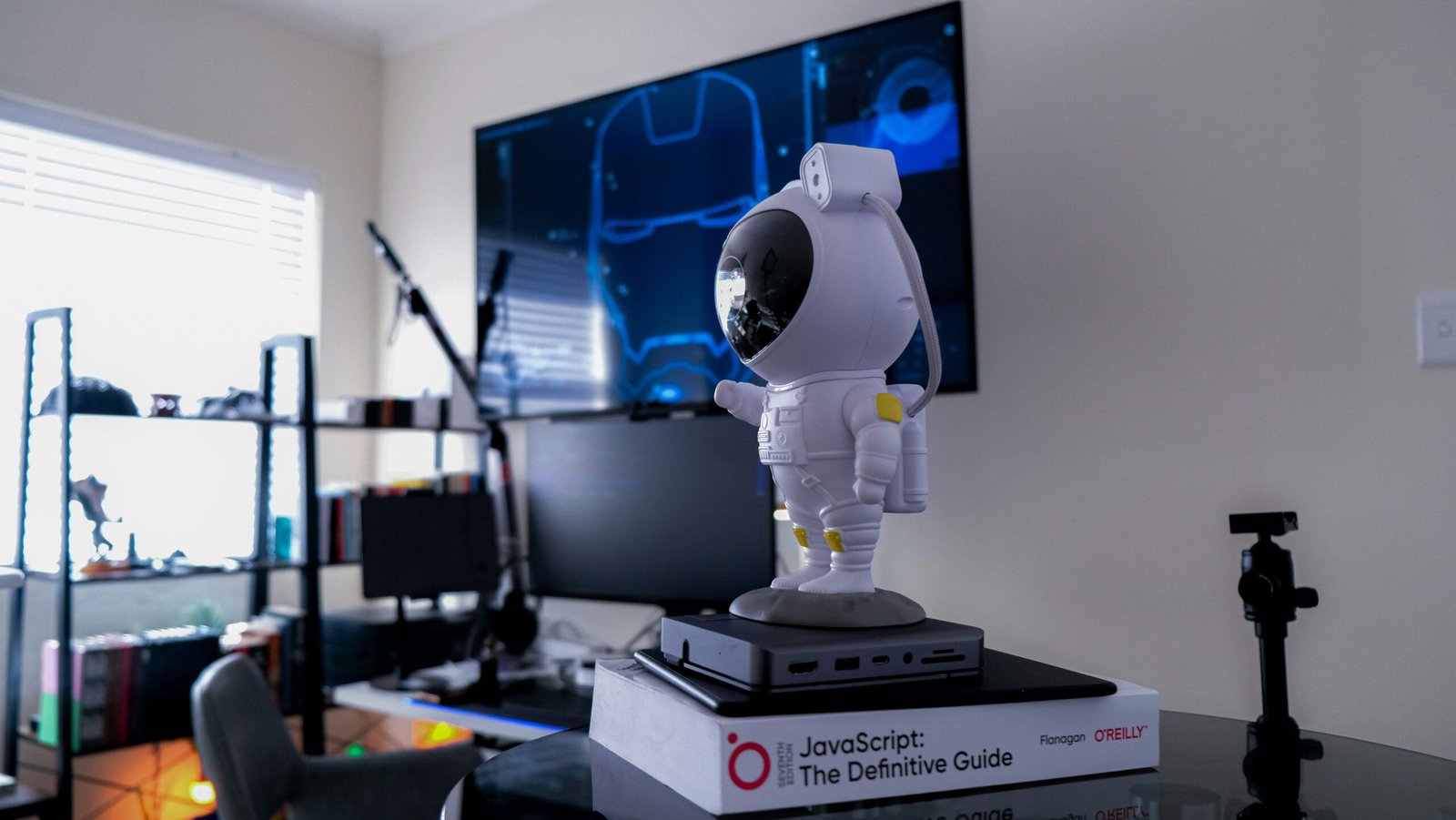
What is a Closure?
Contents
In the realm of JavaScript, closures represent a fundamental concept that plays a pivotal role in how functions operate and interact with their parent scopes. A closure occurs when a function retains access to its lexical scope, allowing for variables defined within that scope to be preserved and utilized even after the function has been executed outside of that initial context. This capability is a crucial aspect of JavaScript, enabling developers to create more dynamic and encapsulated code.
To illustrate this concept, consider the following simple example. Imagine a function called `outerFunction`, which initializes a variable `outerVariable`. Inside this function, a nested function called `innerFunction` is defined, which logs the `outerVariable` to the console. When `outerFunction` is invoked, it returns the `innerFunction`, effectively creating a closure. Even after `outerFunction` has completed execution, calling `innerFunction` will still display the value of `outerVariable`, showcasing the closure’s ability to access data from its enclosing scope.
Understanding closures is essential for JavaScript developers as they enable powerful programming paradigms, like data privacy and partial application of functions. Closures also facilitate the creation of functions with persistent state, which can be used in various scenarios, such as event handling, asynchronous programming, and module patterns. The significance of closures extends beyond mere function definitions; it shapes how developers structure their applications and manage variable scope effectively.
So to speak, closures are a core part of JavaScript: they are what makes the language agile and allows one to encode lexical scoping along with encapsulation. Hence, to master the language and make advanced programming possible, one must completely understand closures.
How Closures Work
In JavaScript, it uses the concept of closures to define the most important aspect of the program. The containment of the environment or scope, where it was defined for functions, is still accessible for that function, even after a return from the outer function. This is comprehensive throughout the lexical scoping model in JavaScript, which explains how variable names are resolved in nested functions. Closing occurs when an inner function maintains references to variables of its outer function, allowing that inner function to reference any such variable in a subsequent invocation.
Behold the example of closures in action through lexical scoping: we could create an outer function named makeCounter, which defines a variable count inside it, and returns an inner function called increment. Invoke increment to increase count and see it printed on the screen. Hence, the function increments count by closure because, even since makeCounter finished executing, increment still has access to count.
function makeCounter() { let count = 0; return function increment() { count++; console.log(count); };}const counter = makeCounter();counter(); // Outputs: 1counter(); // Outputs: 2
In this case, the variable count lives outside the scope of the makeCounter function. Every time the increment function gets called, it will always have a reference to the count, showing how a closure maintains state. Such things are very important in programming, especially with respect to asynchronous programming and private data management.
Closures also provide the execution context through which developers can construct function factories and event handlers and even build IIFEs (Immediately Invoked Function Expressions). Understanding how a closure works takes one not just further in the mastery of JavaScript but also makes optimizing how a code is structured easier without compromising variable space.
Creating Closures in JavaScript
Closures in JavaScript are a powerful feature that allows inner functions to access the scope of their outer functions, creating a persistent environment for variables. To understand how to create closures effectively, consider composing an outer function that defines a variable, followed by an inner function that utilizes that variable. This structure demonstrates the essence of closures.
Here’s a basic example:
function outerFunction() { var outerVariable = "I am outside!"; function innerFunction() { console.log(outerVariable); } return innerFunction;}const closureExample = outerFunction();closureExample(); // Output: I am outside!
In this example, when outerFunction
is invoked, it initializes outerVariable
and then returns innerFunction
. Although outerFunction
has completed execution, the returned innerFunction
retains access to outerVariable
. This is a fundamental characteristic of closures.
Closures are also valuable for creating private variables. Attaining encapsulation can be accomplished through a slightly more complex implementation, as shown below:
function createCounter() { let count = 0; return { increment: function() { count++; return count; }, decrement: function() { count--; return count; }, getCount: function() { return count; } };}const counter = createCounter();console.log(counter.increment()); // Output: 1console.log(counter.increment()); // Output: 2console.log(counter.getCount()); // Output: 2console.log(counter.decrement()); // Output: 1
In this example, count
is a private variable inaccessible from outside. The functions returned by createCounter
are closures that can read and modify the count directly, demonstrating how closures can be employed to manage state effectively while hidden from the global scope.
Use Cases of Closures
Closures in JavaScript serve several practical purposes, significantly enhancing the language’s functionality and flexibility. One primary use case of closures is data encapsulation. By using closures, developers can create functions that “close over” their lexical environment, preserving private variables in a way that is not directly accessible from the global scope. This feature allows for better control over data and enables the implementation of information hiding, which is an essential principle in software engineering. For instance, a function can return another function that accesses private variables, thereby keeping those variables safe from external access.
Another important application of closures is in partial function application. This technique allows developers to fix a few arguments of a function and generate a new function. By leveraging closures, it becomes easy to create specialized functions dynamically. For example, if you have a function that multiplies two numbers, you can establish a closure that fixes one of the numbers, returning a new function that only requires the remaining variable. This approach can lead to simpler and more declarative code, as seen in scenarios involving event handlers in UI programming.
Additionally, closures are invaluable for creating private variables. In JavaScript, due to its prototypal inheritance, there is no built-in way to declare private variables at the object level. However, by utilizing closures, developers can simulate private variables effectively. This method ensures that certain data is not accessible from the outside, thus safeguarding its integrity. For example, through a constructor function, one can define a private variable and expose only selected methods that allow controlled access to that variable. These use cases illustrate how closures can significantly influence coding patterns, foster better design principles, and simplify complex tasks, making them a vital feature of JavaScript programming.
Common Pitfalls with Closures
JavaScript closures are powerful constructs that enable developers to create private variables and functions. However, they can lead to unintended consequences if not understood and implemented properly. One common mistake involves the misuse of closures within loops. When using closures to maintain state in loop iterations, developers may inadvertently capture the variable reference instead of its value. This results in unexpected behavior, as all closures hold a reference to the same variable, leading to outcomes that deviate from the intended logic.
Consider a scenario where a function is defined within a loop to establish event listeners for a series of elements. If the loop variable is captured directly by the closure, every listener will reference the last value of that variable once the loop has completed. To mitigate this issue, a common practice is to utilize an Immediately Invoked Function Expression (IIFE) to create a new scope for each iteration. By doing so, each closure retains its own copy of the variable, resulting in the expected behavior.
Another misconception about closures is their inherent performance cost. While it’s true that closures can consume more memory due to the retention of variables, it is essential to balance this concern with the advantages they provide in terms of encapsulation and functionality. Developers should optimize their use of closures by limiting their scope, avoiding unnecessary variables, and being mindful of how closures are structured. A sound practice is to define closures at the appropriate level, minimizing their longevity when they are no longer needed.
In conclusion, understanding closures is crucial for any JavaScript developer to avoid common pitfalls. Adequate attention to the nuances of variable references in loops and performance considerations can significantly improve the behavior and efficiency of JavaScript code. A comprehensive grasp of these concepts enhances one’s ability to leverage closures effectively, ensuring that they serve their intended purpose without introducing errors or inefficiencies.
Closures and the Event Loop
To comprehend the interaction between closures and JavaScript’s event loop, it is essential to first grasp the concept of the event loop itself. The event loop is a crucial component of JavaScript that manages how asynchronous operations are executed. In JavaScript, operations that take time, such as network requests or timers, are handled asynchronously to prevent blocking the main thread. This allows for a more responsive user experience, enabling the execution of multiple operations simultaneously.
Closures play a significant role in this asynchronous landscape. A closure is created when a function retains access to its lexical scope, even when the function is executed outside that scope. This feature becomes particularly potent within callback functions, where closures can encapsulate the state at the moment the closure is created. As asynchronous events occur, such as a user clicking a button or a timer finishing its countdown, the closure helps preserve the context in which the callback was defined.
For instance, a common scenario involves setting a timeout function that modifies some local variable. Due to the event loop’s handling of asynchronous tasks, the original variables would become inaccessible if not for the closure that encapsulates them. This locks the variable’s state at the time the closure was created, allowing the callback to access those values when the timer expires, regardless of how much time has elapsed. Importantly, this behavior can sometimes lead to unexpected results if not managed properly, as callbacks that reference the same variables will all see the final state after all events have run.
In summary, understanding how closures interact with the event loop is crucial for mastering JavaScript’s asynchronous programming model. It ensures that developers can effectively manage state and behavior in their applications without falling victim to common pitfalls associated with variable scope and callback execution timing.
Closures in Real-world Applications
Closures are a fundamental aspect of JavaScript, providing a unique way to encapsulate and manage variables, especially in the context of frameworks and libraries. One prominent example can be found in jQuery, where closures are frequently employed for event handling. When a jQuery event listener is set up, it often utilizes closures to capture the surrounding state, making sure it retains access to variables at the time the listener was created. For instance, when you define a click event handler in jQuery, any variables defined within the scope of that handler remain accessible even after the handler is invoked, preventing unexpected behavior and ensuring that the appropriate data is referenced.
Moving to modern frameworks such as React, closures play an equally critical role. In React components, especially functional components, closures help manage state and lifecycle methods. When a stateful functional component is created using hooks, closures allow the component to remember the current state and function definitions as they exist at the time of rendering. This is particularly evident with the use of the useEffect hook, where closures can capture props and state, enabling fine-grained control over side effects within the application.
Moreover, closures are essential in implementing various functional programming patterns commonly used in JavaScript. For example, higher-order functions can leverage closures to maintain access to variables across function invocations. When creating a function that generates another function (like a counter function), the inner function maintains a reference to the outer function’s variables through a closure, allowing manipulation of its state across multiple calls without exposing it to the global scope.
This encapsulation of state and behavior is pivotal for writing maintainable and error-free code in the dynamic landscape of JavaScript development. As frameworks and libraries continue to evolve, understanding the use of closures becomes increasingly important for developers looking to harness the full potential of JavaScript.
Performance Considerations when Using Closures
Closures are a powerful feature in JavaScript that allow functions to maintain access to their lexical scope, even when the function is executed outside of that scope. However, while closures provide a range of benefits, they can also have significant performance implications that developers must consider. One of the primary concerns involves memory usage. When a closure is created, it retains references to its outer function’s variables. This can lead to increased memory overhead, especially if many closures are created in a long-running application.
The accumulation of closures can result in memory leaks when they are not properly managed. Each closure holds onto its surrounding variables, preventing those variables from being garbage collected, which can lead to higher memory consumption over time. For applications that demand optimal performance, especially those that run in environments with limited resources, understanding these implications is critical. It may be prudent to avoid using closures in performance-critical sections of the application.
In practical scenarios, developers should carefully analyze the necessity of closures. If a function can achieve the desired outcome without retaining access to outer scope variables, it is generally better to employ simpler function structures. This not only improves performance but also enhances code readability and maintainability. Additionally, utilizing alternatives such as module patterns or revealing module patterns can provide similar encapsulation benefits without the added memory burden that closures impose.
Ultimately, while closures serve essential purposes in JavaScript programming, it is vital to strike a balance between their utility and the system’s performance demands. Developers should weigh the trade-offs and opt for strategies that minimize memory retention where closures are concerned, ensuring that applications remain efficient and responsive.
Conclusion
In reviewing the intricacies of JavaScript closures, it is evident that they play a crucial role in modern web development. We have explored how closures allow for the creation of private variables, enabling developers to encapsulate functionality and maintain state across function invocations. The ability to return inner functions demonstrates how closures can be effectively utilized to create more organized and maintainable code.
Moreover, we delved into scenarios where closures can improve performance and readability, such as in event handlers, callbacks, and managing asynchronous operations. By allowing functions to retain access to their lexical scope, closures introduce a level of memory management that can help prevent potential errors. Understanding these concepts fosters better programming practices and equips developers with the tools needed for more complex coding challenges.
As we have highlighted, experimenting with closures in your coding practices can lead to a greater comprehension of the language’s capabilities. Whether it is through practical implementation in your projects or exploring theoretical applications, the knowledge gained from mastering closures is invaluable. By leveraging closures skillfully, programmers can write cleaner, more efficient JavaScript that adheres to best practices, resulting in more robust applications.
In conclusion, the mastery of JavaScript closures not only enhances your skill set but also contributes to the overall quality of your code. By integrating this understanding into your coding style, you can unlock new potential and elevate your programming projects significantly. Embrace the power of closures and their benefits, and witness the positive impact they can have on your coding journey.